python assignment you need to access for spyder to do the assignment
Task
We are going to create some simple rules for translating normal English into Gibberish. A common rule is to add sounds to each syllable, but since syllables are difficult to detect in a simple program, we’ll use a rule of thumb: every vowel denotes a new syllable. Since we are adding a Gibberish syllable to each syllable in the original words, we must look for the vowels.
To make things more unique, we will have two different Gibberish syllables to add. The first Gibberish syllable will be added to the first syllable in every word, and a second Gibberish syllable will be added to each additional syllable. For example, if our two Gibberish syllables were “ib†and “agâ€, the word “program†would translate to “pribogragam.â€
In some versions of Gibberish, the added syllable depends on the vowels in a word. For example, if we specify “*b†that means we use the vowel in the word as part of the syllable: e.g. “dog†would become “dobog†(inserting “ob†where the “*†is replaced by the vowel “oâ€) and “cat†would become “cabat†(inserting “ab†where “a†is used). Note that the “*†can only appear at the beginning of the syllable (to make your programming easier).
After the Gibberish syllables are specified, prompt the user for the word to translate. As you process the word, make sure you keep track of two things. First, if the current letter is a vowel, add a Gibberish syllable only if the previous letter was not also a vowel. This rule allows us to approximate syllables: translating “weird†with the Gibberish syllable “ib†should become “wibeirdâ€, not “wibeibirdâ€. Second, if we’ve already added a Gibberish syllable to the current word, add the secondary syllable to the remaining vowels. How can you use Booleans to handle these rules?
Finally, print the Gibberish word. Afterwards, ask the user if they want to play again, and make sure their response is an acceptable answer (“yesâ€/“noâ€, “yâ€/“nâ€). Make sure to check the validity for all of your user inputs throughout the program. Don’t let bad input create errors.
Your program will:
- Print a message explaining the game.
- Prompt for two Gibberish syllables (indicate the allowed wildcard character “*â€).
- Prompt for a word to translate.
- Process the word and add the syllables where appropriate.
- Print the final word, and ask if the user wants to play again.
- a)First solve the program using a single Gibberish syllable, without checking for multiple vowels in a row or using the wildcard (“*â€). This means that “weird†with the “ib†syllable will become “wibeibird†(that will not be correct in the final version, but good enough for starting your program: simplify!).
- b)You will need to decide how you will check for vowels. Good possibilities are string indexing or using a Boolean, but use a method that works best with your own program. When you check for vowels it may be handy to create a string vowels = “aeiouAEIOU†and use in vowels to check if a character is a vowel (is the character in the string named vowels).
- c)In this simplified version assume that the Gibberish syllable is exactly two characters long.
- d)For your resulting word start with an empty string and add characters onto it as you process characters from the original word.
Notes and Hints:
You should start with this program, as with all programs, by breaking the program down into parts.
- Getting started: Simplify!
- Now go back and add error checking to make sure the user is entering valid data and that the word entered actually has a usable vowel to make Gibberish out of. Provide user instructions both when starting the program and if you get invalid input (numbers or punctuation in the word). Try to anticipate every possible error a user could make including “kitty at the keyboard.â€
- After that, add the second Gibberish syllable and allow Gibberish syllables longer than two characters.
- a)A Boolean to keep track of whether you have already made a substitution for the first Gibberish syllable will be useful, e.g. done_with_first_vowel = False
- b)I found slicing useful for longer Gibberish syllables.
- Add the wildcard ability after you’ve completed the above steps.
- Finally, add handling of the special case of consecutive vowels. For most people this is the hardest part of the program. It doesn’t add much Python code, but until you see it the logic can be elusive. Try to map it out with a flowchart and pseudocode first. I used a variable named previous_character that held the previous character so when I was looking at a new character I could check whether the previous character had been a vowel.
The string library has a couple of useful tools. If you add import string at the beginning of your program, string.digits and string.ascii_letters are strings that contain all the digits (0 through 9) and all the letters (uppercase and lowercase).
Criteria
Deliverables
- Code: proj05.py – your source code solution (remember to include the date, project number and comments in this file). Be sure to use “proj05.py†for the file name. Be sure to add comments to your code to help me understand it.
- Pseudocode: pseudo05.doc – your pseudocode solution to the problem in a Microsoft Word file. Be sure to use proper indentation and “program†in English (or your first language of choice with an English translation).
- Flowchart: flow05-partX.jpg – your flowchart solution in an image file format (jpg, png, etc.) Be sure to use the proper shapes for each type of statement in your program. Each separate function created should have its own flow image (numbered as flow05-part1.jpg, flow05-part2.jpg, etc.).
Do you need a similar assignment done for you from scratch? We have qualified writers to help you. We assure you an A+ quality paper that is free from plagiarism. Order now for an Amazing Discount!
Use Discount Code "Newclient" for a 15% Discount!
NB: We do not resell papers. Upon ordering, we do an original paper exclusively for you.
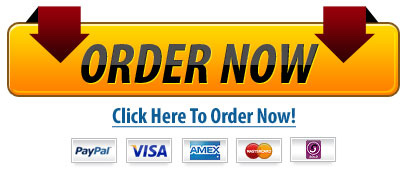